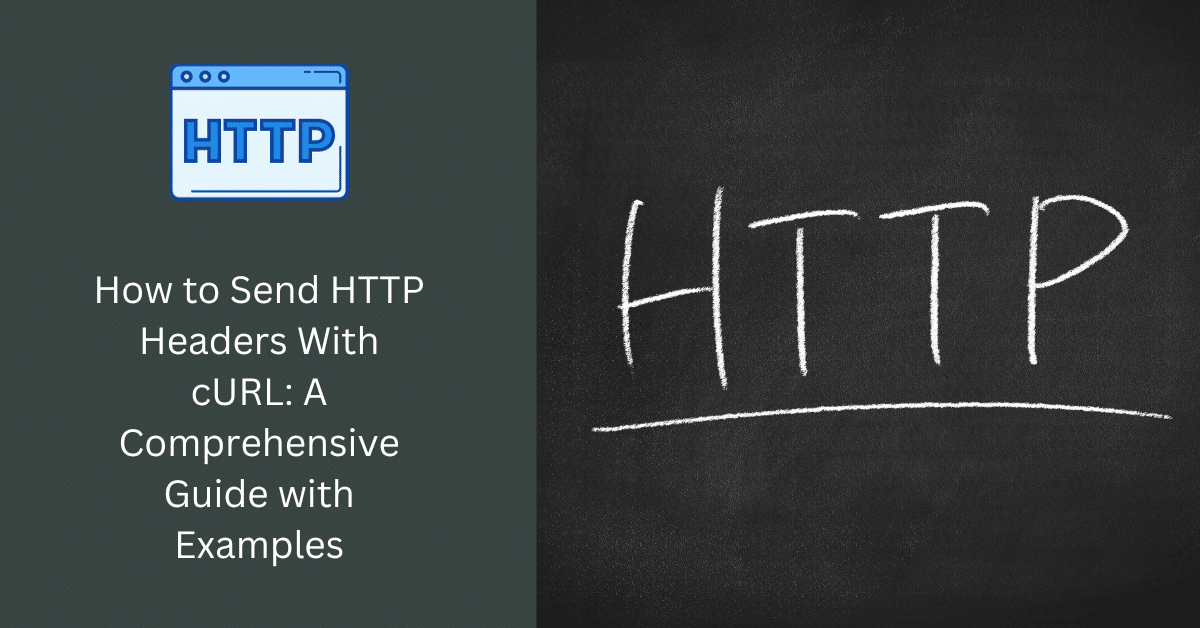
Sending HTTP headers with cURL is a fundamental skill for anyone working with APIs or web services. HTTP headers convey essential information about your request, such as content type, authorization, and more. cURL, a powerful command-line tool for making HTTP requests, allows you to customize and send these headers effortlessly. In this guide, we’ll walk you through the process of sending HTTP headers using cURL, complete with a variety of practical examples.
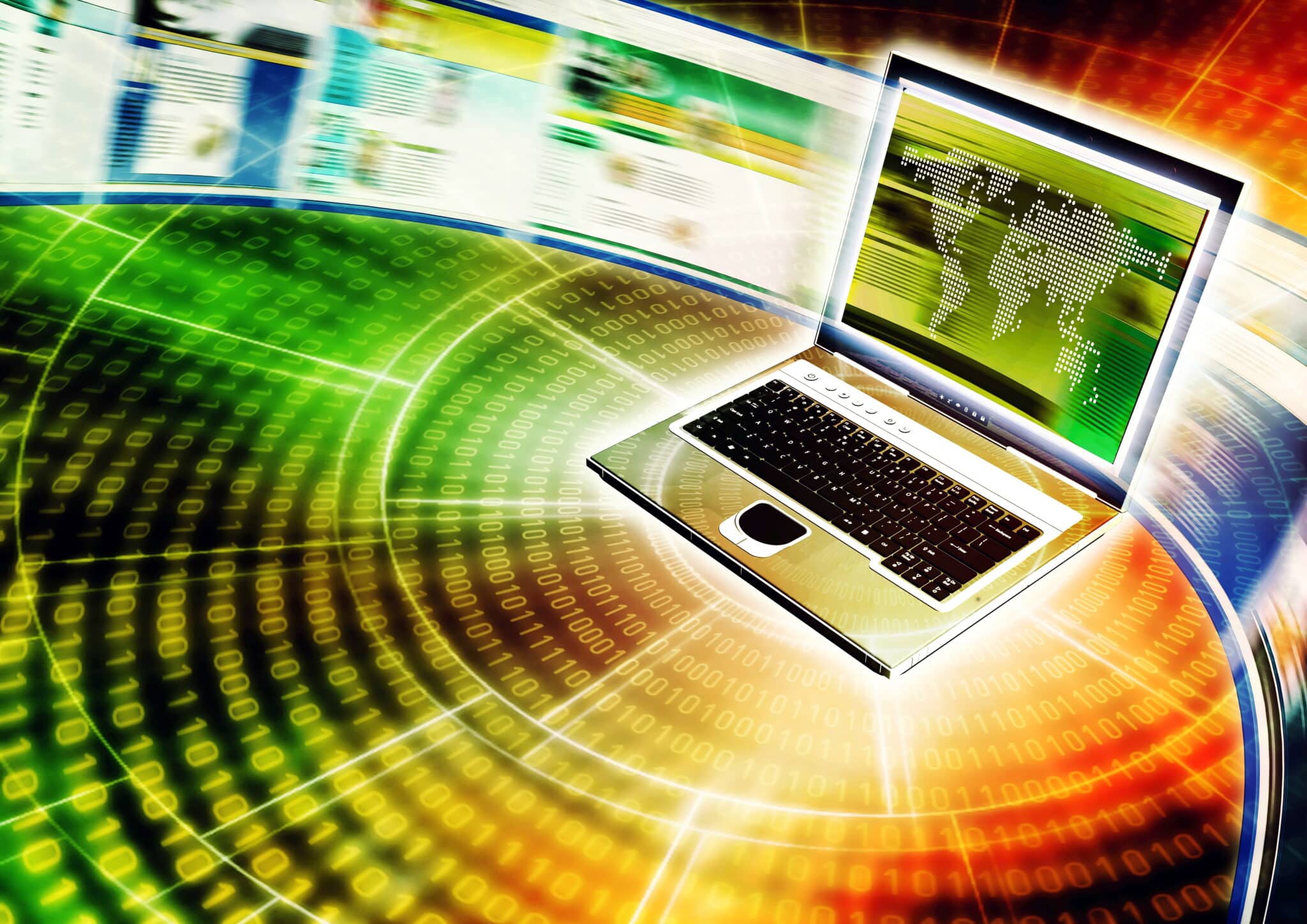
Prerequisites
Before we dive into examples, make sure you have cURL installed on your system. You can check if it’s installed by running:
curl --version
If it’s not installed, you can download it from the official cURL website.
Basic cURL Syntax
The basic syntax for sending an HTTP request with cURL is:
curl [options] [URL]
To include HTTP headers in your request, you can use the -H or –header option followed by the header information.
Example 1: Sending a GET Request with Custom Headers
Let’s start with a simple GET request to a hypothetical API, including a custom header. Suppose you want to include an “Authorization” header with your API key.
curl -H "Authorization: Bearer YOUR_API_KEY" https://api.example.com/resource
Replace YOUR_API_KEY with your actual API key.
Example 2: Sending a POST Request with JSON Data
In this example, we’ll send a POST request with a JSON payload and a custom content type header.
curl -X POST -H "Content-Type: application/json" -d '{"name": "John", "email": "[email protected]"}' https://api.example.com/users
This command specifies the request method as POST (-X POST), sets the content type to JSON (-H “Content-Type: application/json”), and includes the JSON data with -d.
Example 3: Setting Multiple Headers
You can include multiple headers in a single cURL request. Here, we’re sending a request with both “Authorization” and “User-Agent” headers.
curl -H "Authorization: Bearer YOUR_API_KEY" -H "User-Agent: MyClient/1.0" https://api.example.com/resource
Example 4: Sending Cookies
To send cookies with your request, use the -b or –cookie option followed by the cookie data.
curl -b "session=12345; preferences=darkmode" https://api.example.com/dashboard
This example sends two cookies: “session” and “preferences.”
Example 5: Following Redirects
By default, cURL doesn’t follow HTTP redirects. To enable redirect following, use the -L or –location option.
curl -L https://example.com
This command will follow any redirects and display the final page content.
Example 6: Sending Custom User-Agent
You can set a custom User-Agent header to identify your request.
curl -H "User-Agent: MyCustomApp/1.0" https://api.example.com/resource
Custom User-Agent headers are useful when interacting with APIs that require specific user agents.
Example 7: Sending Request Headers Without Data
If you need to send a header without a request body, you can use the -I or –head option.
curl -I https://api.example.com/resource
This command sends a HEAD request, which fetches only the headers and not the actual content.
Example 8: Sending Basic Authentication
To send HTTP Basic Authentication credentials, you can include an “Authorization” header with your username and password encoded in Base64.
curl -H "Authorization: Basic BASE64_ENCODED_CREDENTIALS" https://api.example.com/resource
Replace BASE64_ENCODED_CREDENTIALS with your Base64-encoded username and password (e.g., username:password).
Example 9: Debugging and Verbose Mode
When troubleshooting issues or inspecting the HTTP request and response, you can use cURL’s verbose mode with the -v or –verbose option.
curl -v https://api.example.com/resource
This will display detailed information about the request and response, including headers.
Sending HTTP headers with cURL is a crucial skill for working with web services and APIs. With the examples provided in this guide, you can customize your requests, handle authentication, send cookies, and more. As you become familiar with cURL’s capabilities, you’ll be well-equipped to interact with various web services effectively.
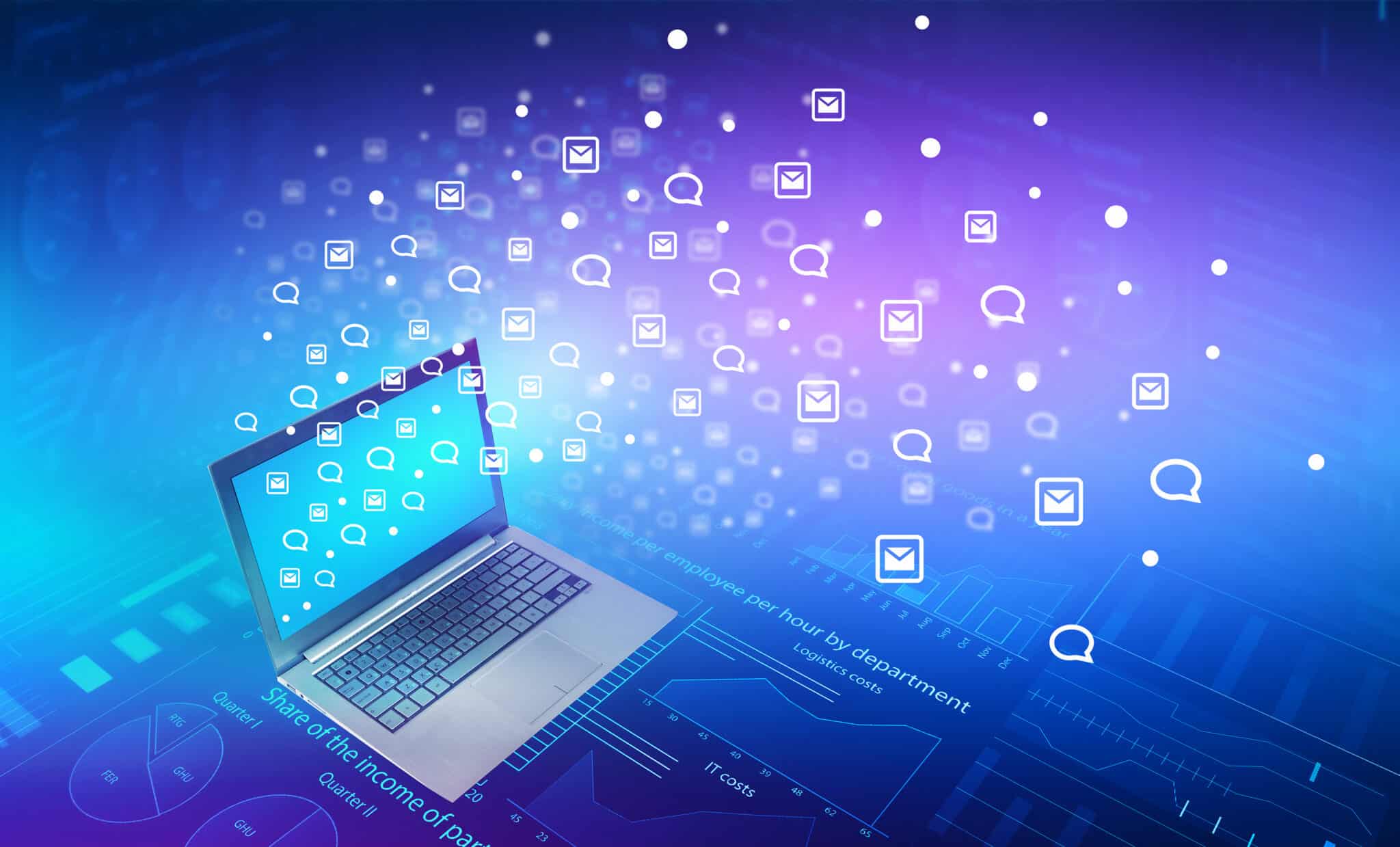
What is cURL, and why would I need to send HTTP headers with it?
cURL is a command-line tool for making HTTP requests to web servers. You might need to send HTTP headers with cURL to provide authentication, specify content types, handle cookies, and customize your requests when interacting with web services or APIs.
How do I include custom headers in a cURL request?
You can include custom headers in a cURL request using the -H or –header option followed by the header information. For example:
curl -H “Authorization: Bearer YOUR_API_KEY” https://api.example.com/resource
Can I send multiple headers in a single cURL request?
Yes, you can send multiple headers in a single cURL request by specifying multiple -H options. For instance:
curl -H “Header1: Value1” -H “Header2: Value2” https://api.example.com/resource
How can I send cookies with my cURL request?
To send cookies with your request, use the -b or –cookie option followed by the cookie data. Here’s an example:
curl -b “session=12345; preferences=darkmode” https://api.example.com/dashboard
How can I view detailed information about my cURL request and response?
To view detailed information, including headers, about your cURL request and response, use the -v or –verbose option. Here’s an example:
curl -v https://api.example.com/resource
These FAQs should help you understand the essentials of sending HTTP headers with cURL and how to perform various tasks like authentication, header customization, and troubleshooting when working with web services and APIs.
What is HTTP Basic Authentication, and how do I send it with cURL?
HTTP Basic Authentication is a method for providing username and password credentials. To send it with cURL, include an “Authorization” header with your Base64-encoded credentials, like this:
curl -H “Authorization: Basic BASE64_ENCODED_CREDENTIALS” https://api.example.com/resource
How can I set a custom User-Agent header in my cURL request?
You can set a custom User-Agent header using the -H option. For example:
curl -H “User-Agent: MyCustomApp/1.0” https://api.example.com/resource
What is the purpose of the -L or –location option in cURL?
The -L or –location option in cURL is used to enable automatic following of HTTP redirects. If a URL redirects to another location, cURL will follow the redirect and fetch the content from the final URL.