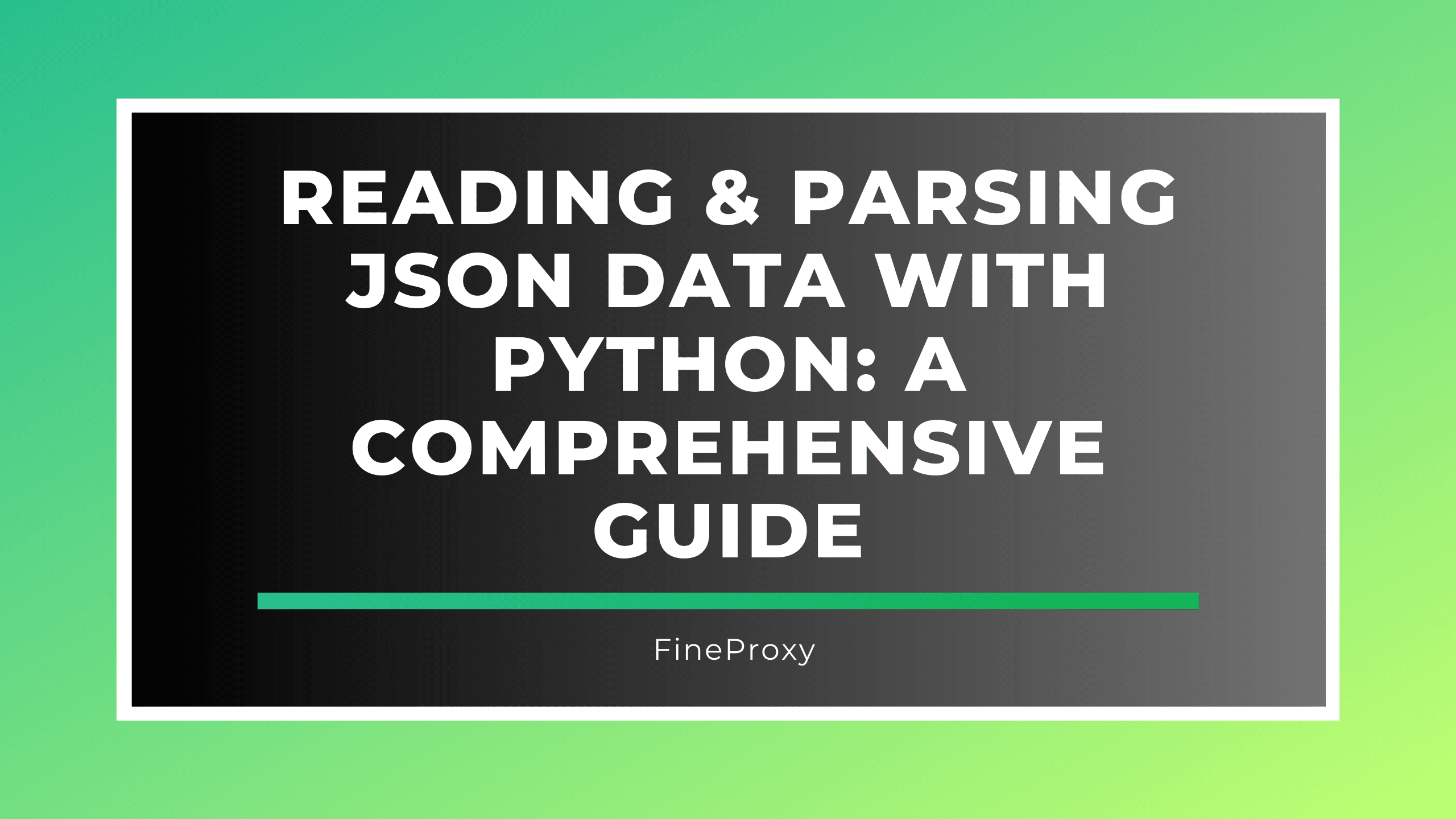
- What is JSON and why is it important in Python programming?
- How can you read JSON data from a file using Python?
- What are the primary functions provided by Python’s
json
module for handling JSON data? - How can large JSON files be handled efficiently in Python?
- What common errors might developers encounter when parsing JSON data with Python?
Table of Contents
The ability to work with JSON (JavaScript Object Notation) data is an essential skill for modern Python developers. JSON is a lightweight data-interchange format that’s easy to read and write for humans, and easy to parse and generate for machines. It is widely used in web development, configuration files, and data interchange between servers and web applications. This guide delves into the process of reading and parsing JSON data with Python, covering the tools, techniques, and tips needed to handle JSON data efficiently.
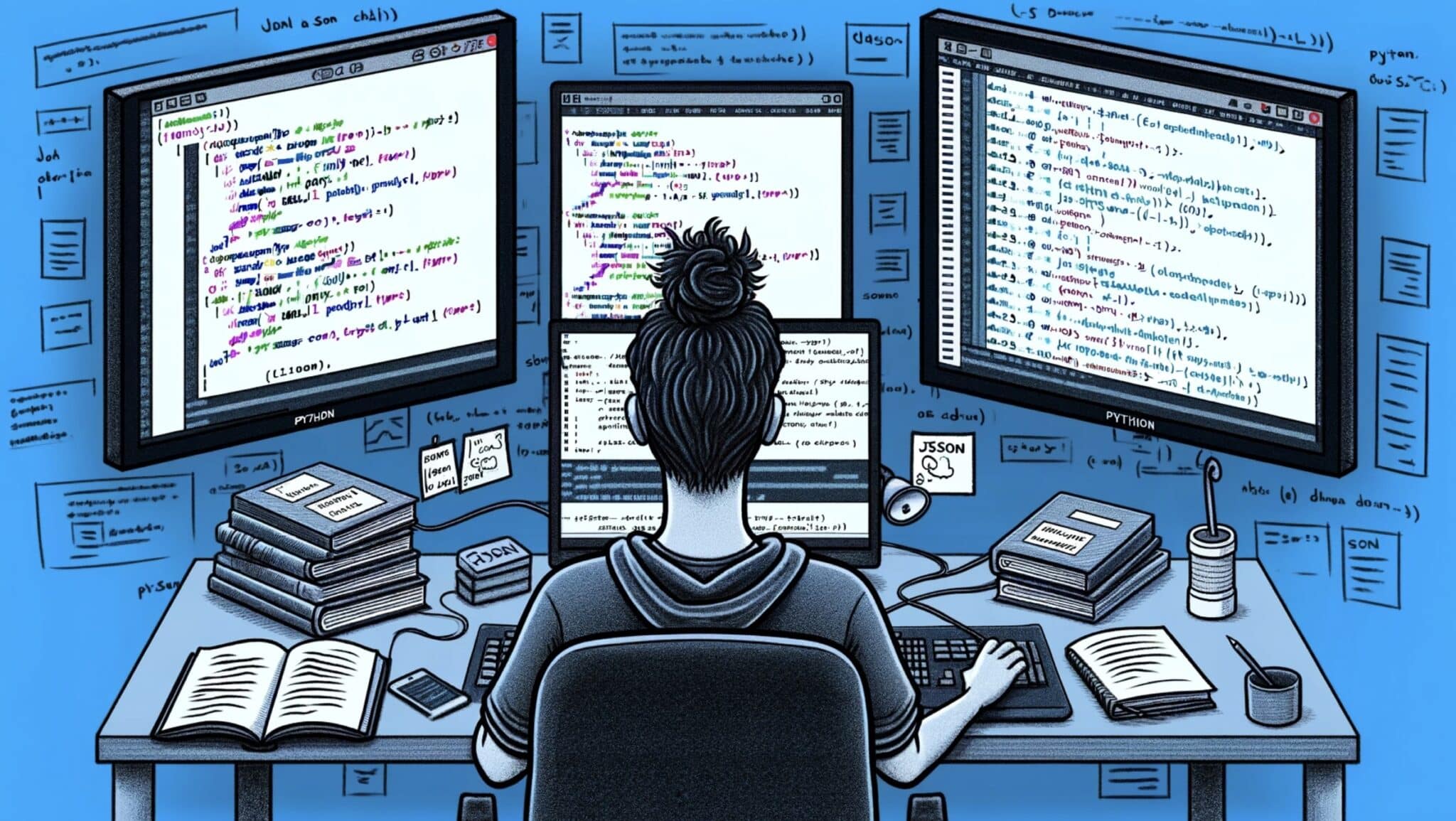
Understanding JSON in Python
Before diving into the specifics of reading and parsing JSON data, it’s crucial to understand what JSON is and why it’s so popular. JSON is structured as a collection of key-value pairs, similar to Python dictionaries, making it a natural fit for data manipulation in Python. Its simplicity and efficiency in representing data structures have made it a preferred choice over XML in many applications.
Tools for Working with JSON in Python
Python provides a built-in module, json
, which is the cornerstone for handling JSON data. This module offers methods to encode and decode JSON data, allowing for easy conversion between JSON strings and Python data structures. Here’s a quick overview of the primary functions provided by the json
module:
json.load()
: Parses JSON data from a file-like object into Python objects.json.loads()
: Parses JSON data from a string into Python objects.json.dump()
: Converts Python objects into JSON data and writes them to a file-like object.json.dumps()
: Converts Python objects into a JSON-formatted string.
Reading JSON Data from a File
To read JSON data from a file, you can use the json.load()
method. This method reads the file, parses the JSON data, and converts it into a Python dictionary or list, depending on the structure of the JSON data. Here’s an example:
import json
with open('data.json', 'r') as file:
data = json.load(file)
This simple code snippet opens the data.json
file, reads its contents, and parses the JSON data into a Python dictionary stored in the variable data
.
Parsing JSON Data from a String
In cases where JSON data is received as a string, such as from a web API, the json.loads()
method is used. This method takes a JSON-formatted string and converts it into a Python object. For example:
import json
json_string = '{"name": "John", "age": 30}'
data = json.loads(json_string)
This code snippet parses the json_string
containing JSON data into a Python dictionary.
Practical Examples of JSON Parsing
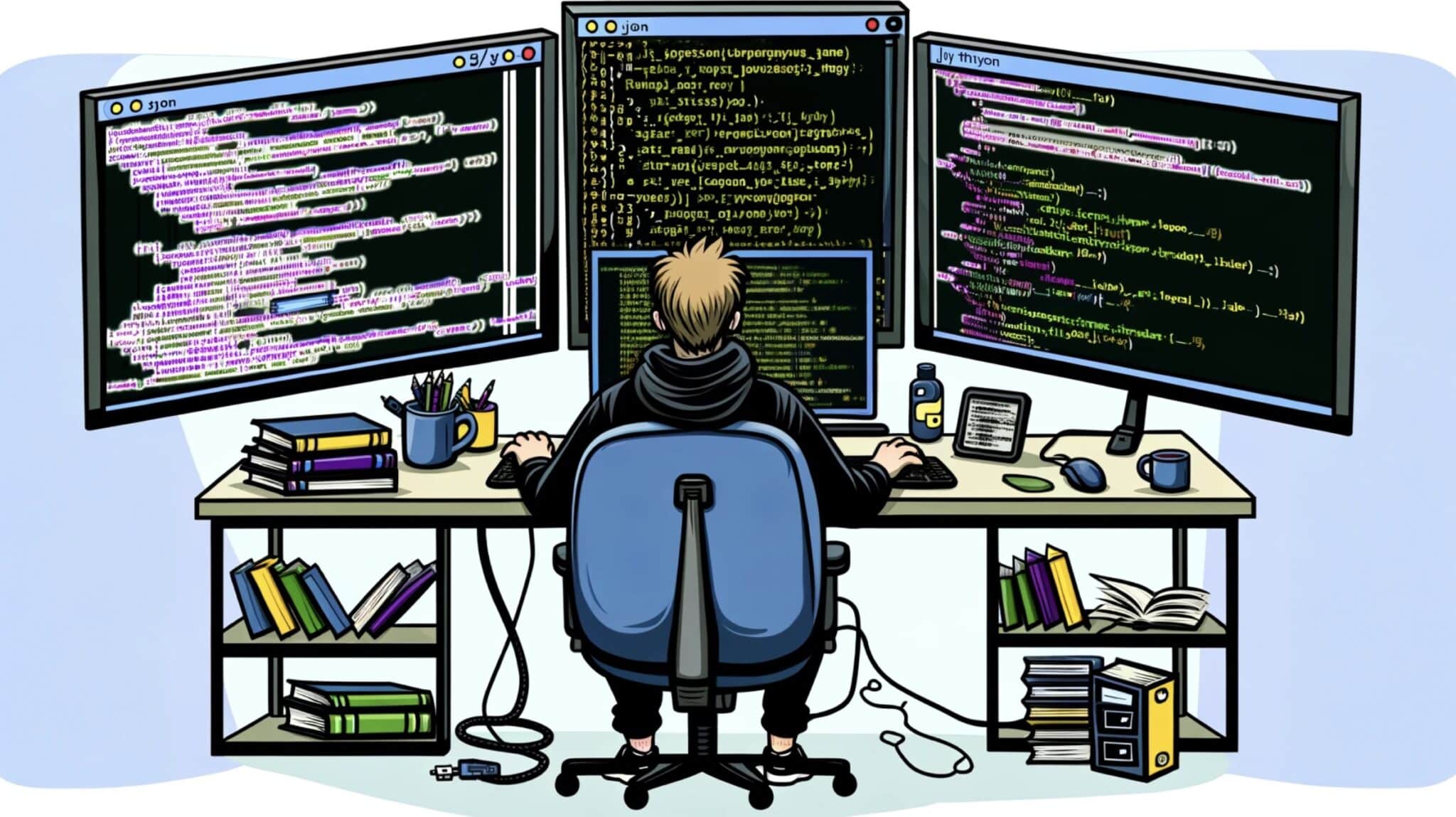
To better understand how JSON parsing works in real-world scenarios, consider the following examples:
- Configuring Web Applications: JSON files are often used for configuring web applications. Parsing these files allows Python scripts to access configuration settings.
- Interacting with Web APIs: Many web APIs return data in JSON format. Using
json.loads()
, this data can be easily converted into Python objects for further manipulation.
Advanced Techniques for JSON Parsing
While the json
module covers most needs, advanced use cases might require additional techniques:
- Handling Large JSON Files: For large JSON files, consider using a streaming approach or libraries like
ijson
that allow for incremental parsing. - Merging JSON Data: In some cases, you might need to merge data from multiple JSON sources. Python’s dictionary update methods can be helpful here.
Common Errors in JSON Data Handling
Even experienced developers can encounter errors when working with JSON data. Common mistakes include:
- Trying to parse JSON data that is not correctly formatted.
- Forgetting to handle exceptions that may arise during parsing.
Conclusion
Reading and parsing JSON data with Python is a fundamental skill for developers in the digital age. By leveraging Python’s built-in json
module and following best practices, developers can efficiently work with JSON data, enabling seamless data interchange and configuration management in web applications.
As you continue to work with JSON data, remember to experiment with different parsing techniques and stay updated on the latest libraries and tools available in the Python ecosystem. With practice and patience, you’ll become adept at handling JSON data in your Python projects.
Comments (0)
There are no comments here yet, you can be the first!