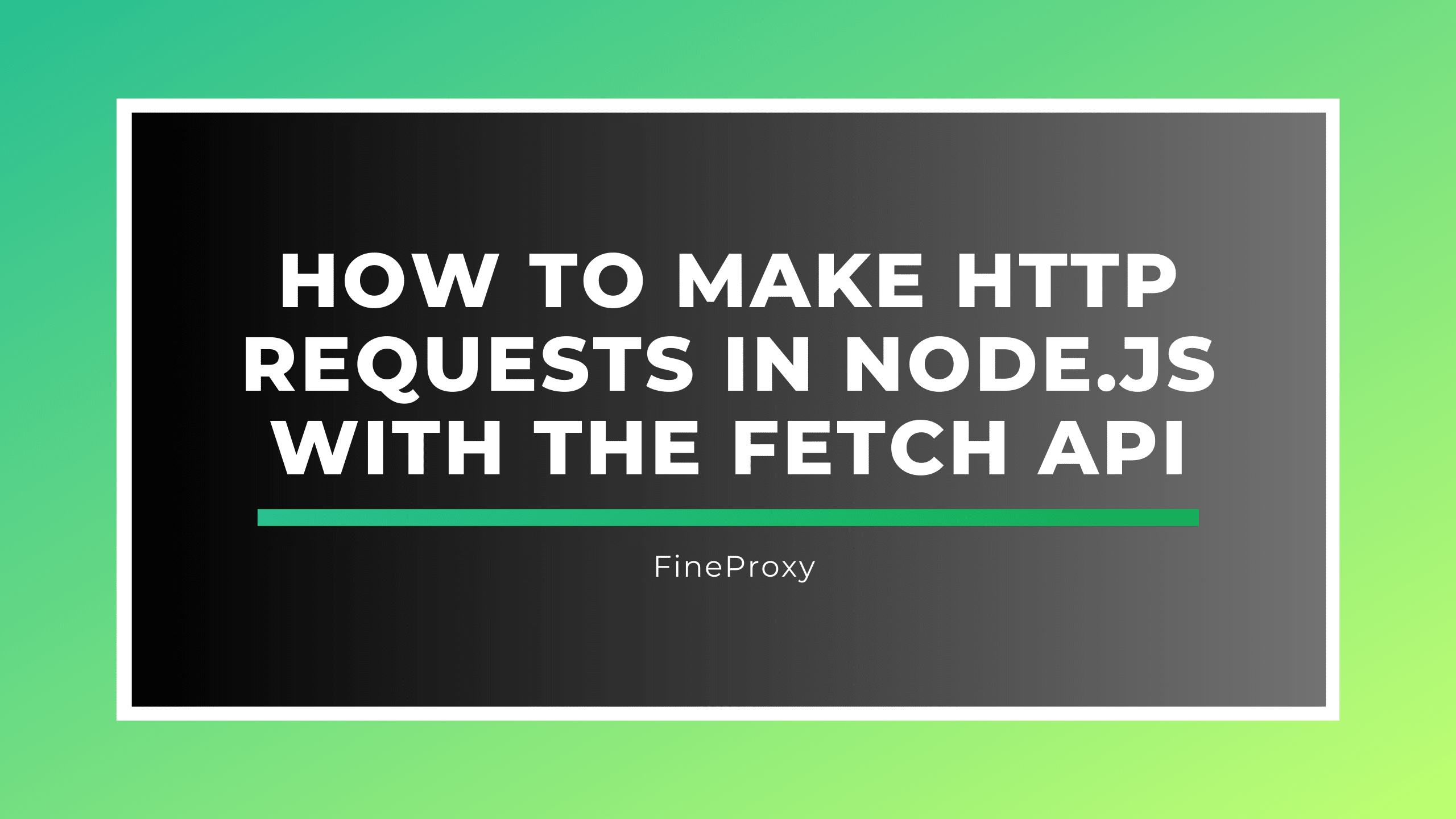
Table of Contents
The ability to make HTTP requests is a foundational aspect of modern web development. In Node.js, the Fetch API has emerged as a popular and powerful tool for this purpose, allowing developers to make asynchronous network requests in a way that is both efficient and easy to understand. This article will guide you through the process of making HTTP requests in Node.js using the Fetch API, covering key concepts, tools, and best practices.
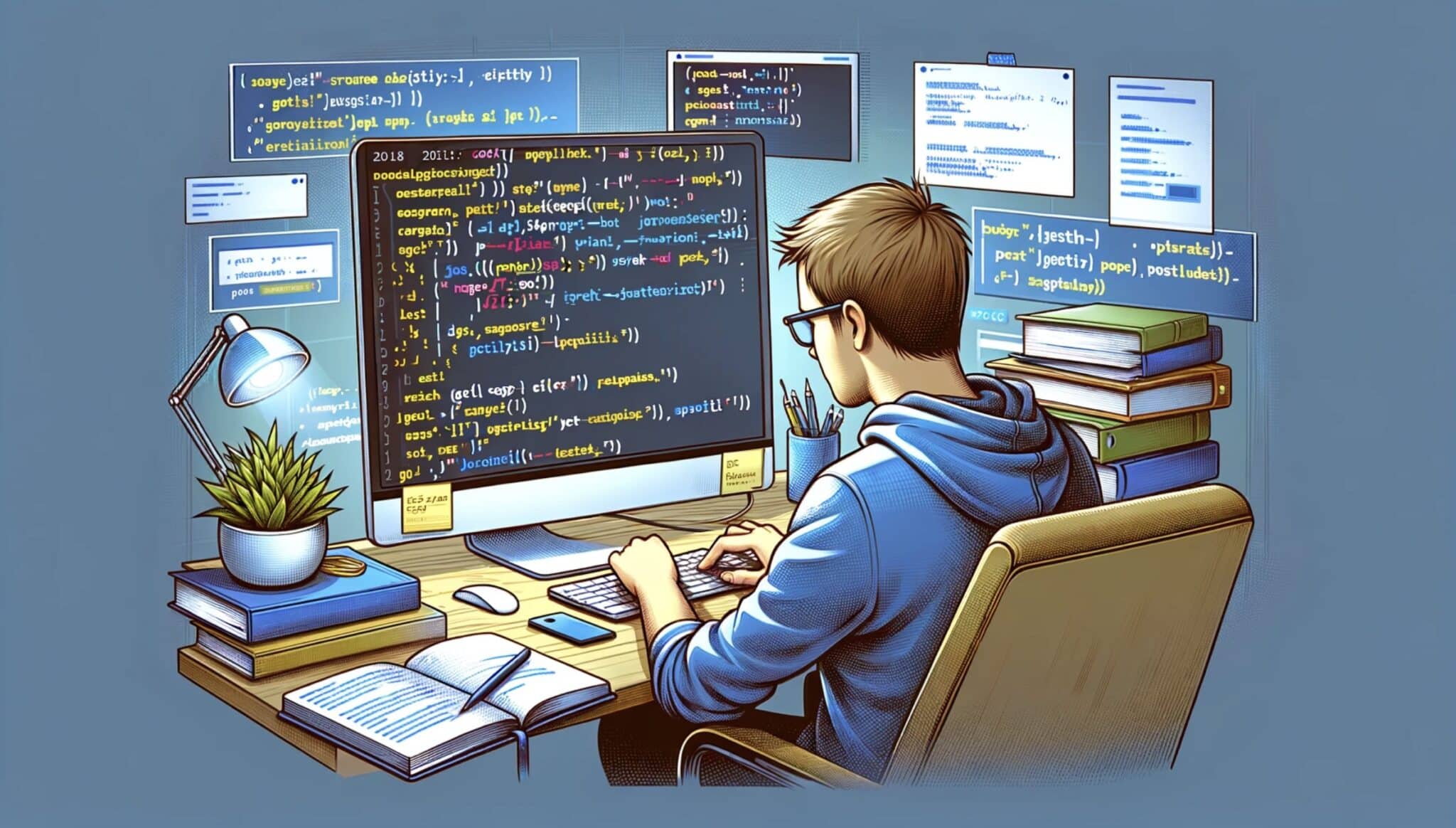
Introduction to the Fetch API in Node.js
The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. Originally designed for the browser, the Fetch API is now available in Node.js through polyfills like node-fetch
. It is promise-based, making it suitable for handling asynchronous operations in a more manageable way.
Why Choose Fetch API for Node.js?
- Simplicity: The Fetch API simplifies the process of making HTTP requests with a cleaner and more readable syntax compared to older methods like XMLHttpRequest (XHR).
- Asynchronous Handling: Thanks to its promise-based nature, the Fetch API enhances asynchronous operations handling, enabling cleaner code through async/await syntax.
- Flexibility: It allows for easy customization of requests with various options for headers, query parameters, and body content.
Setting Up the Fetch API in Your Node.js Project
Before you can start making HTTP requests, you need to set up the Fetch API in your Node.js environment. This involves installing the node-fetch
package, which is a Node.js implementation of the Fetch API.
Installing node-fetch
To install node-fetch
, run the following command in your project directory:
npm install node-fetch
This command adds node-fetch
to your project, allowing you to use the Fetch API in a Node.js environment.
Making HTTP Requests Using the Fetch API
The Fetch API simplifies the process of making various types of HTTP requests, such as GET, POST, PUT, and DELETE. The following sections will cover how to make these requests and handle their responses.
Making a GET Request
GET requests are used to retrieve data from a specified resource. Here’s how you can make a GET request using the Fetch API:
const fetch = require('node-fetch');
async function fetchData(url) {
const response = await fetch(url);
const data = await response.json();
return data;
}
fetchData('https://api.example.com/data')
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Making a POST Request
POST requests are used to send data to a server to create or update a resource. The code snippet below demonstrates how to make a POST request:
const fetch = require('node-fetch');
async function postData(url, data) {
const response = await fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(data),
});
const responseData = await response.json();
return responseData;
}
postData('https://api.example.com/data', { key: 'value' })
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Handling Responses and Errors
When making HTTP requests, it’s important to handle both the responses and any potential errors appropriately.
Handling Responses
The Fetch API returns a promise that resolves into a Response
object. This object contains the response status, headers, and the body. You can use methods like .json()
, .text()
, or .blob()
to parse the response body.
Handling Errors
Error handling with the Fetch API involves checking both network errors and the response status code. Here’s an example of how to handle errors:
fetch(url)
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.catch(error => console.error('There has been a problem with your fetch operation:', error));
Best Practices for Using the Fetch API in Node.js
Adhering to best practices when using the Fetch API can help ensure that your code is efficient, secure, and easy to maintain.
Use Async/Await for Cleaner Code
Leveraging the async/await syntax can make your asynchronous code cleaner and more readable.
Error Handling
Implement comprehensive error handling to manage both network failures and HTTP errors gracefully.
Secure Your Requests
Always ensure that sensitive data is securely transmitted, especially when dealing with APIs over HTTPS.
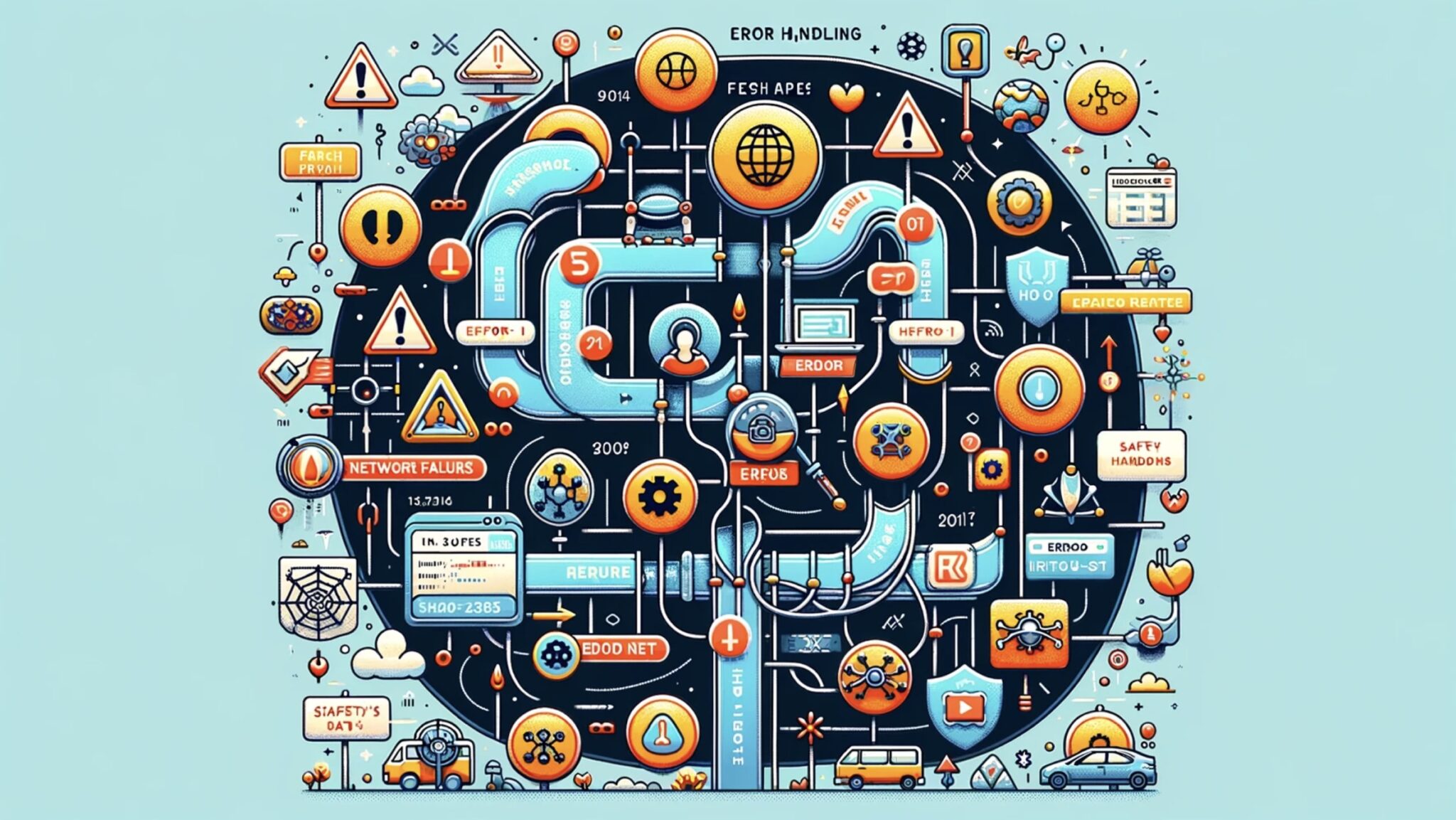
Conclusion
The Fetch API offers a modern, powerful, and flexible way to make HTTP requests in Node.js. By understanding how to set up and use the Fetch API, as well as following best practices for request handling and error management, developers can build robust and efficient web applications. Whether you’re retrieving data from an API, submitting form data, or interacting with external services, the Fetch API provides the tools you need to perform these tasks with ease and precision.