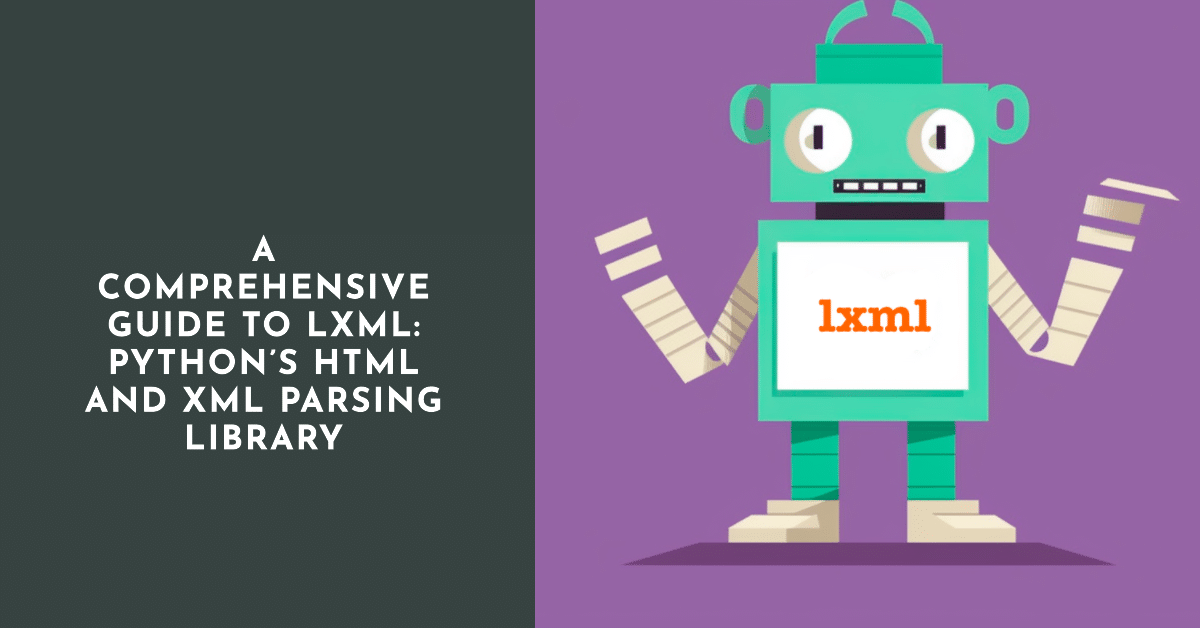
lxml is a Python library used for parsing XML and HTML documents. It combines the speed and XML feature completeness of libxml2 and libxslt with the simplicity of a native Python API, making it a go-to tool for web scraping and data extraction from XML and HTML sources. This article provides an in-depth look at lxml, exploring its features, use cases, advantages, and installation process.
Understanding lxml
lxml is a powerful library, yet it is easy to use and accessible even to beginners in Python programming. lxml leverages the API of libxml2 and libxslt, providing comprehensive support for XML, XPath, XSLT, XML Schema, RELAX NG, and more.
Installing lxml
To install lxml, you can use pip, the Python package installer. Here’s how you can do it:
pip install lxml
Remember that you may need to use pip3 instead of pip or use a virtual environment, depending on your Python setup.
Parsing XML and HTML with lxml
One of the primary uses of lxml is to parse XML and HTML documents. Parsing is the process of analyzing a string of symbols, either in natural language or in computer languages, according to the rules of a formal grammar.
XML Parsing
To parse XML with lxml, you can use the etree module:
from lxml import etree
xml_data = """
<root>
<element key="value">text</element>
</root>
"""
root = etree.fromstring(xml_data)
print(root.tag) # output: root
print(root[0].tag) # output: element
print(root[0].text) # output: text
print(root[0].get("key")) # output: value
HTML Parsing
Similarly, to parse HTML documents, lxml provides the html module:
from lxml import html
html_data = """
<html>
<body>
<h1>Hello, lxml!</h1>
</body>
</html>
"""
root = html.fromstring(html_data)
print(root.tag) # output: html
print(root[0].tag) # output: body
print(root[0][0].tag) # output: h1
print(root[0][0].text) # output: Hello, lxml!
-
What is lxml?
lxml is a Python library for parsing XML and HTML documents. It combines the speed and XML feature completeness of libxml2 and libxslt with the simplicity of a native Python API.
-
How can I install lxml?
You can install lxml using pip, the Python package installer, with the command pip install lxml.
-
How can I parse XML with lxml?
To parse XML with lxml, you can use the etree module and the fromstring function, which converts an XML string into an Element object that you can work with.
-
How can I parse HTML with lxml?
Similar to XML parsing, lxml provides the html module for parsing HTML documents. You can use the fromstring function to convert an HTML string into an Element object.
-
Why should I use lxml instead of other parsing libraries?
lxml is particularly powerful due to its combination of speed and completeness. It offers a simple Pythonic API, making it easy to use while still providing all the features and speed of libxml2 and libxslt.
-
Is lxml better than BeautifulSoup?
The choice between lxml and BeautifulSoup depends on the specific requirements of the task, your familiarity with the libraries, and personal preference.
lxml:
lxml is generally faster and more memory-efficient than BeautifulSoup. If performance is a critical factor, lxml might be the better choice.
lxml supports XPath queries, which can be more powerful and flexible than the CSS-style selectors used in BeautifulSoup.
The lxml API closely follows the standard Pythonic API for XML and HTML manipulation, making it intuitive for those already familiar with Python’s xml module.BeautifulSoup:
BeautifulSoup can handle poorly formed HTML or XML documents better than lxml. If you’re dealing with “messy” or malformed data, BeautifulSoup might be the better choice.
BeautifulSoup’s API is considered by some to be more user-friendly than lxml’s, making it a popular choice for beginners or those prioritizing ease of use over speed.
BeautifulSoup has a very active community, which can be a boon for finding help or resources.
In conclusion, neither lxml nor BeautifulSoup is objectively better than the other; it really depends on the specifics of the project and the user’s preferences. It can be helpful to experiment with both to see which one fits your use-case and coding style better.
Here are some trustworthy resources where you can learn more about lxml and XML/HTML parsing:
- lxml Official Documentation: The official documentation is always the best place to start. It provides a comprehensive overview of the library, including installation instructions, tutorials, and API reference.
- Python 101: An Intro to lxml: This article provides a beginner-friendly introduction to lxml.
- Web Scraping with Python and lxml: A DataCamp community tutorial that demonstrates how to use lxml for web scraping.
- libxml2 and libxslt Official Documentation: Since lxml is based on these libraries, their official documentation can be useful for understanding the underlying mechanics.
- Python lxml tutorial on TutorialsPoint: This tutorial covers lxml basics and demonstrates some practical web scraping tasks.