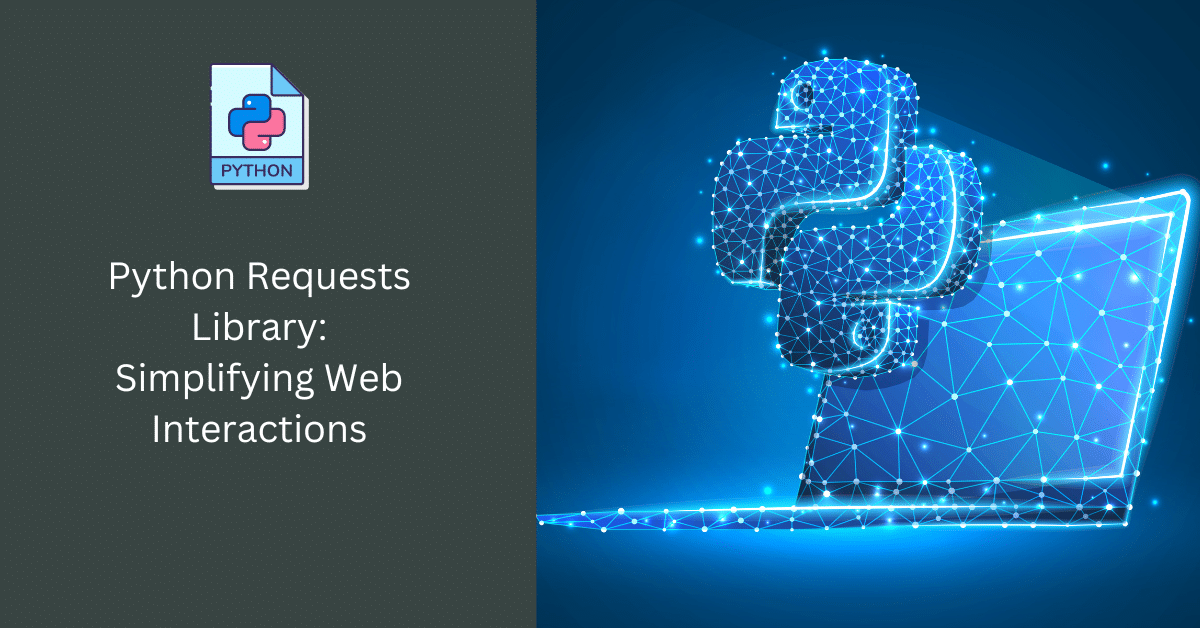
In today’s digital age, accessing web resources and APIs is an integral part of software development. Python, a versatile programming language, offers various libraries to simplify web interactions. One such indispensable tool is the Python Requests library. In this article, we’ll explore what the Python Requests library is, how to use it effectively, and why it’s a must-have for any Python developer.
Introduction to Python Requests
The Python Requests library is a popular third-party library that simplifies sending HTTP requests and handling responses. It abstracts the complexities of making HTTP requests, providing an easy-to-use API for Python developers.
Installing the Requests Library
Before we dive into using the library, you’ll need to install it. You can do this using pip, the Python package manager:
pip install requests
Making Your First HTTP Request
Let’s start with the basics. To make an HTTP GET request using Python Requests, you can use the following code:
import requests response = requests.get('https://example.com')
HTTP Methods
Python Requests supports various HTTP methods. Two commonly used methods are GET and POST.
GET Requests
GET requests are used to retrieve data from a server. They are the most common type of HTTP request.
response = requests.get('https://api.example.com/data')
POST Requests
POST requests are used to send data to a server. They are often used for submitting forms or uploading files.
data = {'key': 'value'} response = requests.post('https://api.example.com/submit', data=data)
Working with Query Parameters
You can include query parameters in your requests to filter or customize the data you receive from a server.
params = {'search': 'Python', 'category': 'programming'} response = requests.get('https://api.example.com/search', params=params)
Headers and Authentication
Python Requests allows you to set custom headers and handle various authentication methods easily.
headers = {'User-Agent': 'MyApp/1.0'} response = requests.get('https://api.example.com/resource', headers=headers) # Basic Authentication response = requests.get('https://api.example.com/secure', auth=('username', 'password'))
Handling Responses
Once you’ve made a request, you need to work with the response. You can access the response content, headers, and more.
print(response.text) # Get response content as text print(response.headers) # Get response headers print(response.status_code) # Get the HTTP status code
Session Management
Sessions allow you to persist certain parameters across multiple requests, such as cookies and authentication.
session = requests.Session() session.get('https://api.example.com/login') # Subsequent requests within the session will retain session data.
Timeouts and Retries
You can set timeouts to limit the maximum time a request should take. Additionally, Requests supports automatic retries on failed requests.
requests.get('https://api.example.com/resource', timeout=5, retries=3)
Error Handling
Proper error handling is crucial when dealing with web requests. Python Requests provides ways to handle exceptions gracefully.
try: response = requests.get('https://api.example.com/resource') response.raise_for_status() # Raise an exception for HTTP errors except requests.exceptions.HTTPError as err: print(f"HTTP error: {err}")
Best Practices
To ensure clean and efficient code, follow best practices such as using constants for URLs and keeping your code modular.
Common Use Cases
Explore various real-world use cases where Python Requests can simplify web interactions.
Security Considerations
Learn about security measures like verifying SSL certificates and avoiding potential vulnerabilities.
Performance Optimization
Discover tips for optimizing the performance of your web requests, including connection pooling.
In conclusion, the Python Requests library is a powerful tool for simplifying web interactions in your Python projects. Whether you’re fetching data, sending data, or interacting with web APIs, Requests makes it easy and efficient.
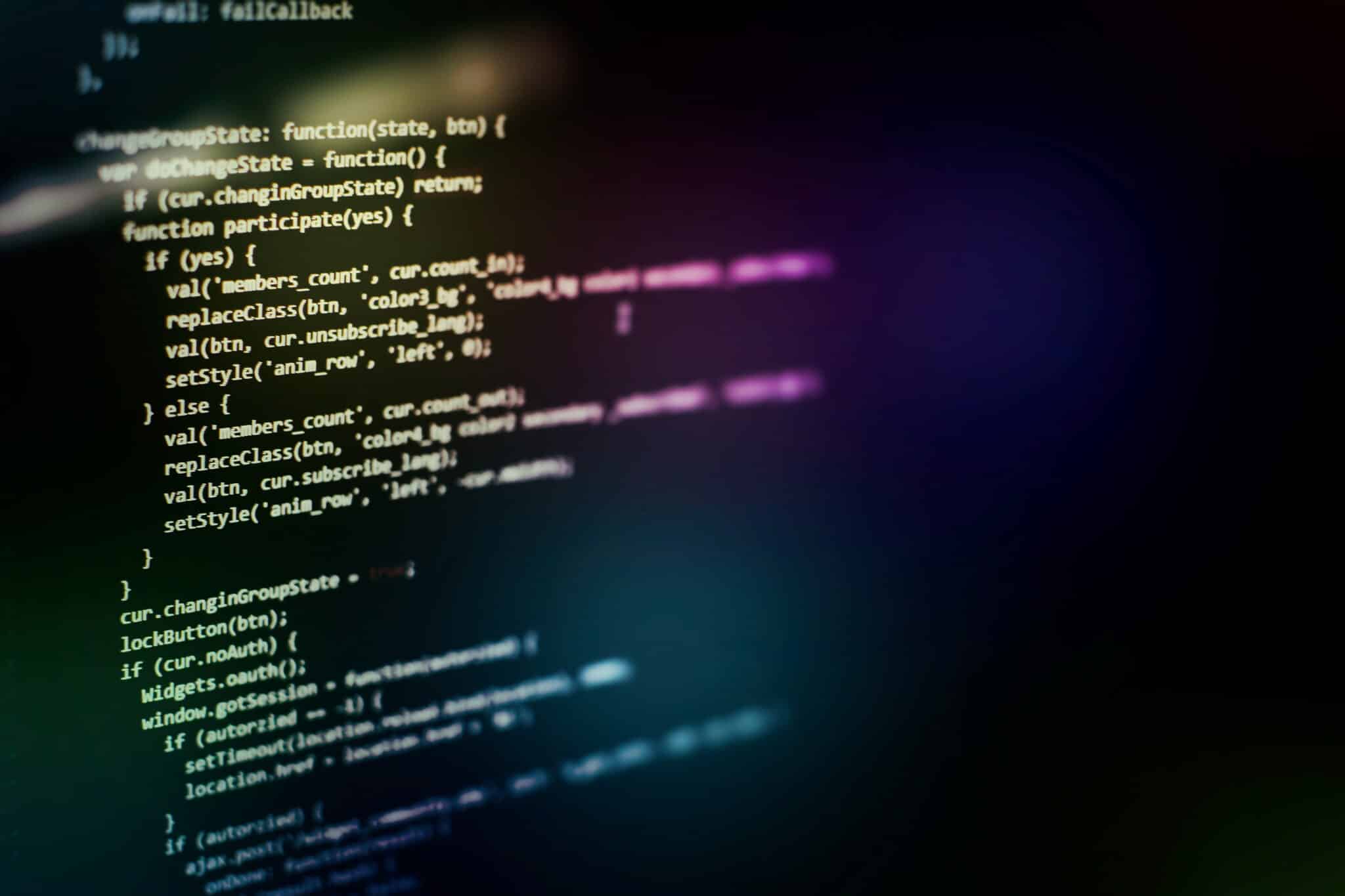
Comments (0)
There are no comments here yet, you can be the first!